5. Consume the loader
Picking the right method
We recommend consuming the loader with either withLoader
or AwaitLoader
.
- Use
withLoader
if you want to use the loader to affect the whole component. - Use
AwaitLoader
if you want to use the loader in a more conditional way, or if you only want a small section of your component to start loading data, after the rest of the component has rendered.
You could also use useLoader
, which is the hook that withLoader
and AwaitLoader
use under the hood. This will simply return the aggregated queries of the loader, and is useful if you want to use the data, but not the loading and error states of the loader.
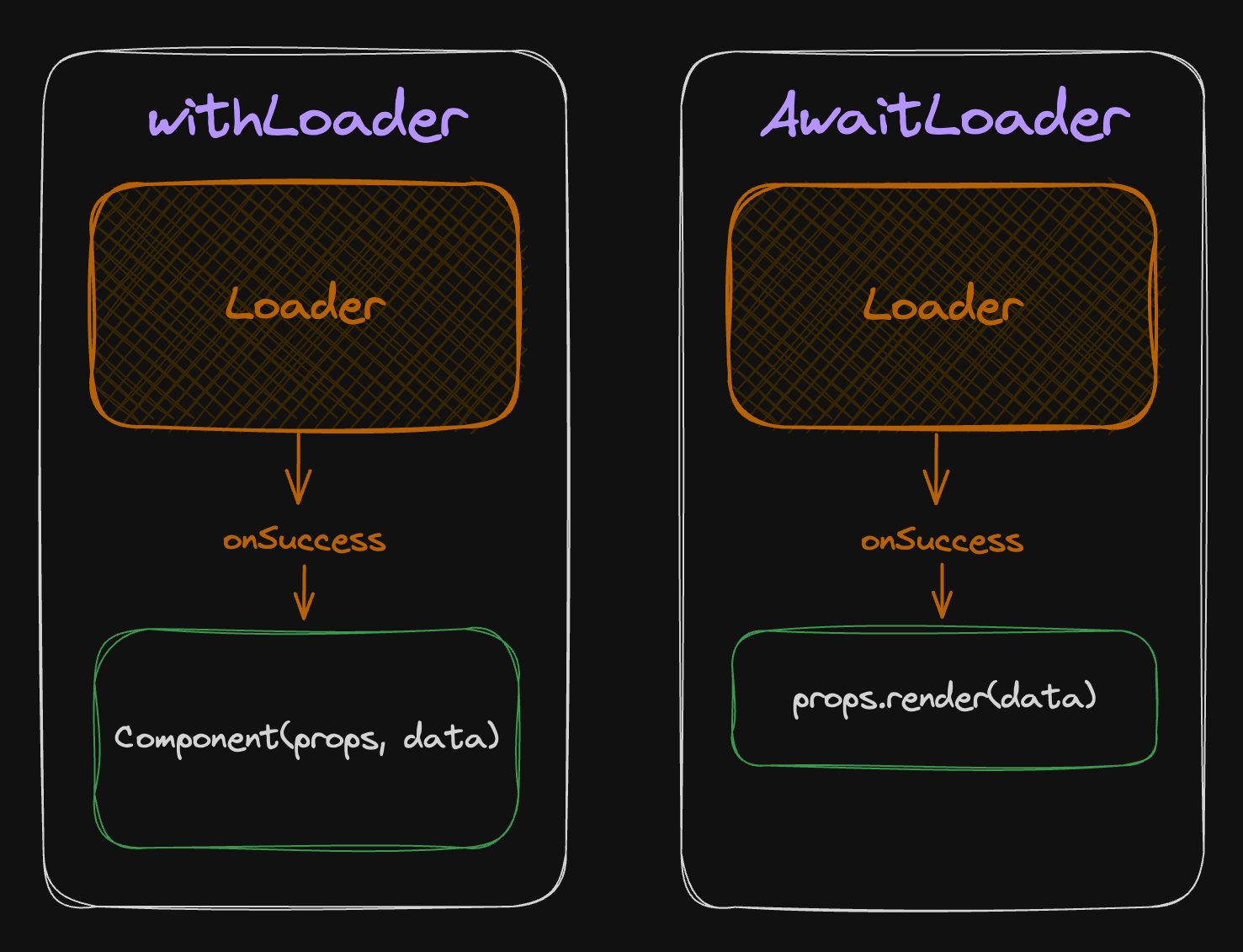
Examples
Select a tab below to see an example of how you would consume the loader using that specific method.
- withLoader
- <AwaitLoader>
- useLoader
A convenient wrapper that ensures that the component is only rendered when it has data.
This is the recommended and optimal way to consume the loaders.
import { withLoader } from "@ryfylke-react/rtk-query-loader";
import { userRouteLoader } from "../loaders/baseLoader";
type Props = {
/* ... */
};
export const UserRoute = withLoader((props: Props, loader) => {
// `queries` is typed correctly, and ensured to have loaded.
const {
user,
posts
} = loader.queries;
return (
<article>
<header>
<h2>{user.data.name}</h2>
{user.isFetching || posts.isFetching ? (<InlineLoading />) : null}
</header>
<main>
{posts.data.map((post) => (...))}
</main>
</article>
);
}, userRouteLoader);
New in version 1.0.4
If you prefer not using higher order components, or want to use the loader in a more conditional way, you can use the <AwaitLoader
/> component.
import { AwaitLoader } from "@ryfylke-react/rtk-query-loader";
import { userRouteLoader } from "./baseLoader";
const UserRoute = () => {
return (
<AwaitLoader
loader={userRouteLoader}
render={({ queries }) => (
<article>
<header>
<h2>{queries.user.data.name}</h2>
</header>
<main>
{queries.posts.data.map((post) => (...))}
</main>
</article>
)}
/>
);
};
Every Loader
contains an actual hook that you can call to run all the queries and aggregate their statuses as if they were just one joined query.
This is convenient if you want to simply use the data, but not the loading and error states of the loader.
import { userRouteLoader } from "./baseLoader";
const useLoaderData = userRouteLoader.useLoader;
type Props = {
/* ... */
};
const UserRoute = (props: Props) => {
const loaderQuery = useLoaderData();
if (loaderQuery.isLoading) {
// ...
}
if (loaderQuery.isError) {
// ...
}
// ...
};